Up and coding with Next.js, React & AdminLTE¶
Installation and setup¶
Tip
- Double click on code blocks to select all text within in.
- Replace NEW_PROJECT_NAME='NextProject' with your project name so you can following along.
- You can copy and paste all the commands below straight into your terminal to create all the files as required as you go along.
# Set your project name
NEW_PROJECT_NAME='NextProject'
# Traverse to your project directory
cd ~/Sites
# Init our project (the directory will be created for you)
mkdir $NEW_PROJECT_NAME
cd $NEW_PROJECT_NAME
# Create our package.json
yarn init -y
# Add next, react and react-dom
yarn add next react react-dom
# Make our pages directory
mkdir pages
# Open our project in webstorm
wstorm .
{
"scripts": {
"dev": "next",
"build": "next build",
"start": "next start"
}
}
Add a index.js page in our pages directory.
cat <<'-EOF' > pages/index.js
import "../styles/styles.scss"
export default () => (<h1>Hello next.js</h1>);
-EOF
Run yarn run dev to boot the next.js server and open localhost:3000 in your browser to see the application. As you update the code it should automatically reload, also note the page is being rendered entirely on the server.
Add SASS¶
We’ll use SASS as we’re using bootstrap 4 with AdminLTE v3. Use the @zeit/next-sass package to help with this.
yarn add @zeit/next-sass node-sass
# Install via node
# npm install --save @zeit/next-sass node-sass
cat <<'-EOF' > next.config.js
const withSass = require('@zeit/next-sass')
module.exports = withSass()
-EOF
mkdir styles
cat <<'-EOF' > styles/styles.scss
$color: blue;
h1 {
color: $color;
}
-EOF
Create pages/_document.js¶
We create our own custom _document.js to extend the one provided by next for customization.
cat <<'-EOF' > pages/_document.js
import Document, { Head, Main, NextScript } from 'next/document'
export default class MyDocument extends Document {
render() {
return (
<html>
<Head>
<link rel="stylesheet" href="/_next/static/style.css" />
</Head>
<body>
<Main />
<NextScript />
</body>
</html>
)
}
}
-EOF
Run Next.js¶
We can now run next.js using the scripts we specified earlier.
yarn run dev
Open your browser to http://localhost:3000.
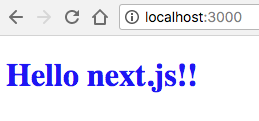
You should see this, the styling is done by our css preprocessor.
Install AdminLTE¶
Lets add AdminLTE and reference it in our application.
yarn add admin-lte@v3 bootstrap
# npm install add admin-lte@v3 bootstrap --save
mkdir static
mkdir styles
cat <<'-EOF' > styles/styles.scss
/**
* App entry scss file using bootstrap and AdminLTE v2
*
* @url https://getbootstrap.com/docs/4.0/getting-started/theming/
* @url https://getbootstrap.com/docs/4.0/getting-started/webpack/#importing-precompiled-sass
*/
@import '~bootstrap/scss/bootstrap';
@import "~admin-lte/build/scss/AdminLTE-raw.scss";
$color: blue;
h1 {
color: $color;
}
-EOF
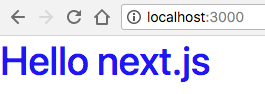
The font color is blue from styles.scss and the font style from AdminLTE.
Add a Header, Sidebar & Content Component¶
We need to add a header, sidebar and content. Let’s do that next.
Note
- There’s a lot of code here because we create the components in different files, it’s just HTML.
- Double click on code blocks to select all the text, then copy and paste it into your terminal (whilst in the root of your app) and it will create all the files for you.
cp node_modules/admin-lte/dist/js/adminlte.js static/
cp node_modules/admin-lte/dist/js/adminlte.js.map static/
cp node_modules/admin-lte/dist/js/adminlte.min.js static/
cp node_modules/admin-lte/dist/js/adminlte.min.js.map static/
Update _document.js to include our libraries¶
We’ll include the layout libraries via regular script tags, we’ll also add font-awesome, jquery and bootstrap.
cat <<'-EOF' > pages/_document.js
import Document, {Head, Main, NextScript} from 'next/document'
export default class MyDocument extends Document {
render() {
return (
<html>
<Head>
<title>My Next.js Project</title>
<link rel="stylesheet" href="/_next/static/style.css"/>
<link href="https://stackpath.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css" rel="stylesheet"/>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"/>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.12.9/umd/popper.min.js"/>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.1.1/js/bootstrap.min.js"/>
{/* This provides all admin-lte functionality - we copied the files to our static directory above */}
<script src="/static/adminlte.js"/>
</Head>
<body className="sidebar-mini">
<Main/>
<NextScript/>
</body>
</html>
)
}
}
-EOF
mkdir -p components/Layout
Create Layout Components¶
cat <<'-EOF' > components/Layout/AdminContent.js
import PropTypes from 'prop-types';
const AdminContent = (props) => {
return <div className="content-wrapper" style={{minHeight: '93vh'}}>
<div className="content-header">
{props.title && <div className="container-fluid">
<div className="row mb-2">
<div className="col-sm-10">
<h1 className="m-0 text-dark">{props.title}</h1>
</div>
<div className="col-sm-2 text-right text-muted">
{props.titleButton && props.titleButton}
</div>
</div>
</div>}
</div>
<div className="content">
<div className="container-fluid">
{props.children}
</div>
</div>
</div>
};
AdminContent.propTypes = {
title: PropTypes.string,
titleButton: PropTypes.element,
};
export default AdminContent;
-EOF
cat <<'-EOF' > components/Layout/AdminControlSidebar.js
const AdminControlSidebar = (props) => {
return <aside className="control-sidebar control-sidebar-dark">
<div className="p-3">
<h5>Title</h5>
<p>Sidebar content</p>
</div>
</aside>
};
export default AdminControlSidebar;
-EOF
cat <<'-EOF' > components/Layout/AdminFooter.js
import PropTypes from 'prop-types';
const AdminFooter = (props) => {
if (!props.leftContent && !props.rightContent) {
return null;
}
return <footer className="main-footer clearfix">
{props.rightContent && <div className="float-right d-none d-sm-inline">{props.rightContent}</div>}
{props.leftContent && props.leftContent}
</footer>
};
AdminFooter.propTypes = {
leftContent: PropTypes.element,
rightContent: PropTypes.string,
};
export default AdminFooter;
-EOF
cat <<'-EOF' > components/Layout/AdminHeader.js
import Link from 'next/link';
const AdminHeader = (props) => {
return <nav className="main-header navbar navbar-expand bg-white navbar-light border-bottom">
<ul className="navbar-nav">
<li className="nav-item">
<a className="nav-link" data-widget="pushmenu" href="#"><i className="fa fa-bars"/></a>
</li>
<li className="nav-item d-none d-sm-inline-block">
<Link href="/"><a className="nav-link">Home</a></Link>
</li>
</ul>
<form className="form-inline ml-3">
<div className="input-group input-group-sm">
<input className="form-control form-control-navbar" type="search" placeholder="Search" aria-label="Search"/>
<div className="input-group-append">
<button className="btn btn-navbar" type="submit">
<i className="fa fa-search"/>
</button>
</div>
</div>
</form>
<ul className="navbar-nav ml-auto">
<li className="nav-item dropdown">
<a className="nav-link" data-toggle="dropdown" href="#">
<i className="fa fa-comments-o fa-fw"/>
<span className="badge badge-danger navbar-badge">3</span>
</a>
<div className="dropdown-menu dropdown-menu-lg dropdown-menu-right">
<a href="#" className="dropdown-item">
<div className="media">
<i className="fa fa-comments-o fa-fw mr-3"/>
<div className="media-body">
<h3 className="dropdown-item-title">Brad Diesel<span className="float-right text-sm text-warning"><i className="fa fa-star"/></span></h3>
<p className="text-sm">Call me whenever you can, we have much work to complete over the next few days!</p>
<p className="text-right text-sm text-muted"><i className="fa fa-clock-o"/> 4 Hours Ago</p>
</div>
</div>
</a>
<div className="dropdown-divider"/>
<a href="#" className="dropdown-item dropdown-footer">See All Messages</a>
</div>
</li>
<li className="nav-item dropdown">
<a className="nav-link" data-toggle="dropdown" href="#">
<i className="fa fa-bell-o"/>
<span className="badge badge-warning navbar-badge">15</span>
</a>
<div className="dropdown-menu dropdown-menu-lg dropdown-menu-right">
<span className="dropdown-header">15 Notifications</span>
<div className="dropdown-divider"/>
<a href="#" className="dropdown-item">
<i className="fa fa-envelope mr-2"/> 4 new messages
<span className="float-right text-muted text-sm">3 mins</span>
</a>
<div className="dropdown-divider"/>
<a href="#" className="dropdown-item dropdown-footer">See All Notifications</a>
</div>
</li>
<li className="nav-item">
<a className="nav-link" data-widget="control-sidebar" data-slide="true" href="#"><i className="fa fa-th-large"/></a>
</li>
</ul>
</nav>
};
export default AdminHeader;
-EOF
cat <<'-EOF' > components/Layout/AdminLayoutHoc.js
import "../../styles/styles.scss"
import AdminHeader from "../../components/Layout/AdminHeader";
import AdminSidebar from "../../components/Layout/AdminSidebar";
import AdminControlSidebar from "../../components/Layout/AdminControlSidebar";
import AdminContent from "../../components/Layout/AdminContent";
import PropTypes from 'prop-types';
/**
* Main admin layout - A Higher Order Component
*/
class AdminLayoutHoc extends React.Component {
render() {
return <div className="wrapper">
<AdminHeader/>
<AdminSidebar/>
<AdminContent title={this.props.contentTitle} titleButton={this.props.contentTitleButton}>
{this.props.children}
</AdminContent>
<AdminControlSidebar/>
{/*<AdminFooter rightContent={'Some text for the footer'} leftContent={<div>I must be an element</div>}/>*/}
</div>
}
}
AdminLayoutHoc.propTypes = {
contentTitle: PropTypes.string,
contentTitleButton: PropTypes.element,
};
export default AdminLayoutHoc
-EOF
cat <<'-EOF' > components/Layout/AdminSidebar.js
import Link from 'next/link';
import PropTypes from 'prop-types';
import { withRouter } from 'next/router';
class AdminSidebar extends React.Component {
render() {
const { pathname } = this.props.router;
return <aside className="main-sidebar sidebar-dark-primary elevation-4" style={{minHeight: '846px'}}>
<Link href="/">
<a className="brand-link text-center">
<i className="fa fa-home fa-2x brand-image ml-2"/>
<span className="brand-text font-weight-light">{this.props.projectName && this.props.projectName}</span>
</a>
</Link>
<div className="sidebar">
<nav className="mt-2">
<ul className="nav nav-pills nav-sidebar flex-column" data-widget="treeview" role="menu" data-accordion="false">
<li className="nav-item">
<Link href="/">
<a className={['nav-link', pathname === '/' ? 'active' : ''].join(' ')}>
<i className="nav-icon fa fa-home"/>
<p>Home</p>
</a>
</Link>
</li>
<li className="nav-item">
<Link href="/users">
<a className={['nav-link', pathname === '/users' ? 'active' : ''].join(' ')}>
<i className="nav-icon fa fa-user-circle"/>
<p>
Users
<span className="right badge badge-success">2</span>
</p>
</a>
</Link>
</li>
<li className="nav-item has-treeview menu-closed">
<a href="#" className="nav-link">
<i className="nav-icon fa fa-dashboard"/>
<p>
Other Pages
<i className="right fa fa-angle-left"/>
</p>
</a>
<ul className="nav nav-treeview">
<li className="nav-item">
<a href="#" className="nav-link">
<i className="fa fa-circle-o nav-icon"/>
<p>Active Page</p>
</a>
</li>
<li className="nav-item">
<a href="#" className="nav-link">
<i className="fa fa-circle-o nav-icon"/>
<p>Inactive Page</p>
</a>
</li>
</ul>
</li>
</ul>
</nav>
</div>
</aside>
}
}
AdminSidebar.propTypes = {
projectName: PropTypes.string,
};
AdminSidebar.defaultProps = {
projectName: 'AdminLTE 3'
};
export default withRouter(AdminSidebar)
-EOF
Create and update pages¶
cat <<'-EOF' > pages/index.js
import AdminLayoutHoc from '../components/Layout/AdminLayoutHoc';
export default class Index extends React.Component {
render() {
return <AdminLayoutHoc contentTitle={'Home'} contentTitleButton={<i className="fa fa-2x fa-home"/>} url={this.props.url}>
<div className="row">
<div className="col-md-6">
<div className="card">
<div className="card-header">
<h3 className="card-title">
<i className="fa fa-text-width"/> Headlines
</h3>
<div className="card-tools">
<button type="button" className="btn btn-tool" data-widget="collapse" data-toggle="tooltip" title="Collapse">
<i className="fa fa-minus"/>
</button>
<button type="button" className="btn btn-tool" data-widget="remove" data-toggle="tooltip" title="Remove">
<i className="fa fa-times"/>
</button>
</div>
</div>
<div className="card-body">
<h1>h1. Bootstrap heading</h1>
<h2>h2. Bootstrap heading</h2>
<h3>h3. Bootstrap heading</h3>
<h4>h4. Bootstrap heading</h4>
<h5>h5. Bootstrap heading</h5>
<h6>h6. Bootstrap heading</h6>
</div>
<div className="card-footer text-right text-muted">
<small>Card Footer</small>
</div>
</div>
</div>
<div className="col-md-6">
<div className="card">
<div className="card-header">
<h3 className="card-title">
<i className="fa fa-text-width"/> Text Emphasis
</h3>
</div>
<div className="card-body">
<p className="lead">Lead to emphasize importance</p>
<p className="text-success">Text green to emphasize success</p>
<p className="text-info">Text aqua to emphasize info</p>
<p className="text-primary">Text light blue to emphasize info (2)</p>
<p className="text-danger">Text red to emphasize danger</p>
<p className="text-warning">Text yellow to emphasize warning</p>
<p className="text-muted">Text muted to emphasize general</p>
</div>
</div>
</div>
</div>
<div className="row">
<div className="col-md-6">
<div className="card">
<div className="card-header">
<h3 className="card-title">
<i className="fa fa-text-width"/> Description
</h3>
</div>
<div className="card-body">
<dl>
<dt>Description lists</dt>
<dd>A description list is perfect for defining terms.</dd>
<dt>Euismod</dt>
<dd>Vestibulum id ligula porta felis euismod semper eget lacinia odio sem nec elit.</dd>
<dd>Donec id elit non mi porta gravida at eget metus.</dd>
<dt>Malesuada porta</dt>
<dd>Etiam porta sem malesuada magna mollis euismod.</dd>
</dl>
</div>
</div>
</div>
<div className="col-md-6">
<div className="card">
<div className="card-header">
<h3 className="card-title">
<i className="fa fa-text-width"/> Description Horizontal
</h3>
</div>
<div className="card-body">
<dl className="dl-horizontal">
<dt>Description lists</dt>
<dd>A description list is perfect for defining terms.</dd>
<dt>Euismod</dt>
<dd>Vestibulum id ligula porta felis euismod semper eget lacinia odio sem nec elit.</dd>
<dd>Donec id elit non mi porta gravida at eget metus.</dd>
<dt>Felis euismod semper eget lacinia</dt>
<dd>Fusce dapibus, tellus ac cursus commodo, tortor mauris condimentum nibh, ut fermentum massa justo
sit amet risus.
</dd>
</dl>
</div>
</div>
</div>
</div>
<div className="row">
<div className="col-sm-12">
<div className="card card-default color-palette-box">
<div className="card-header">
<h3 className="card-title">
<i className="fa fa-tag"/> Color Palette
</h3>
</div>
<div className="card-body">
<div className="row">
<div className="col-sm-4 col-md-2">
<h4 className="text-center">Primary</h4>
<div className="color-palette-set">
<div className="bg-primary disabled color-palette"><span>Disabled</span></div>
<div className="bg-primary color-palette"><span>#3c8dbc</span></div>
</div>
</div>
<div className="col-sm-4 col-md-2">
<h4 className="text-center">Info</h4>
<div className="color-palette-set">
<div className="bg-info disabled color-palette"><span>Disabled</span></div>
<div className="bg-info color-palette"><span>#00c0ef</span></div>
</div>
</div>
<div className="col-sm-4 col-md-2">
<h4 className="text-center">Success</h4>
<div className="color-palette-set">
<div className="bg-success disabled color-palette"><span>Disabled</span></div>
<div className="bg-success color-palette"><span>#00a65a</span></div>
</div>
</div>
<div className="col-sm-4 col-md-2">
<h4 className="text-center">Warning</h4>
<div className="color-palette-set">
<div className="bg-warning disabled color-palette"><span>Disabled</span></div>
<div className="bg-warning color-palette"><span>#f39c12</span></div>
</div>
</div>
<div className="col-sm-4 col-md-2">
<h4 className="text-center">Danger</h4>
<div className="color-palette-set">
<div className="bg-danger disabled color-palette"><span>Disabled</span></div>
<div className="bg-danger color-palette"><span>#f56954</span></div>
</div>
</div>
<div className="col-sm-4 col-md-1">
<h4 className="text-center">Gray</h4>
<div className="color-palette-set">
<div className="bg-gray disabled color-palette"><span>Disabled</span></div>
<div className="bg-gray color-palette"><span>#d2d6de</span></div>
</div>
</div>
<div className="col-sm-4 col-md-1">
<h4 className="text-center">Black</h4>
<div className="color-palette-set">
<div className="bg-black disabled color-palette"><span>Disabled</span></div>
<div className="bg-black color-palette"><span>#111111</span></div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</AdminLayoutHoc>
}
}
-EOF
cat <<'-EOF' > pages/users.js
import AdminLayoutHoc from "../components/Layout/AdminLayoutHoc";
import Link from 'next/link';
export default class Users extends React.Component {
render() {
return <AdminLayoutHoc
contentTitle={'Users'}
contentTitleButton={<Link href="/add-user">
<button type="button" className="btn btn-outline-success btn-sm"><i className="fa fa-user-plus fa-fw"/> Add a new user</button>
</Link>}
url={this.props.url}
>
<div className="row">
<div className="col-12">
<div className="card">
<div className="card-header">
<h3 className="card-title">Current active users</h3>
<div className="card-tools">
<div className="input-group input-group-sm" style={{width: '150px'}}>
<input type="text" name="table_search" className="form-control float-right" placeholder="Search"/>
<div className="input-group-append">
<button type="submit" className="btn btn-default"><i className="fa fa-search"/></button>
</div>
</div>
</div>
</div>
<div className="card-body table-responsive p-0">
<table className="table table-hover">
<tbody>
<tr>
<th>ID</th>
<th>User</th>
<th>Date</th>
<th>Status</th>
<th>Reason</th>
<th>Progress</th>
</tr>
<tr>
<td>183</td>
<td>John Doe</td>
<td>11-7-2014</td>
<td><span className="badge bg-success">Approved</span></td>
<td>This user was approved by <b>Anil</b></td>
<td className="align-middle">
<div className="progress progress-xs">
<div className="progress-bar bg-success" style={{width: '100%'}}/>
</div>
</td>
</tr>
<tr>
<td>175</td>
<td>Mike Doe</td>
<td>11-7-2014</td>
<td><span className="badge bg-danger">Denied</span></td>
<td>This user was declined by <b>Anil</b></td>
<td className="align-middle">
<div className="progress progress-xs">
<div className="progress-bar bg-danger" style={{width: '25%'}}/>
</div>
</td>
</tr>
</tbody>
</table>
</div>
</div>
</div>
</div>
</AdminLayoutHoc>
}
}
-EOF
cat <<'-EOF' > pages/add-user.js
import AdminLayoutHoc from "../components/Layout/AdminLayoutHoc";
import Link from 'next/link';
export default class AddUser extends React.Component {
render() {
return <AdminLayoutHoc contentTitle={'Add User'} contentTitleButton={<i className="fa fa-2x fa-user-plus"/>} url={this.props.url}>
<div className="col-md-6 offset-md-3">
<div className="card">
<div className="card-header">
<h3 className="card-title">New User Form</h3>
</div>
<form className="form-horizontal">
<div className="card-body">
<div className="form-group">
<label htmlFor="validation-ex" className="col-sm-3">First name</label>
<div className="col-sm-12">
<input type="text" className="form-control is-valid" id="validation-ex" placeholder="Anil" value="" required/>
<div className="valid-feedback"><i className="fa fa-check-circle"/> Looks good!</div>
</div>
</div>
<div className="form-group">
<label htmlFor="validation-ex" className="col-sm-3">Last name</label>
<div className="col-sm-12">
<input type="text" className="form-control is-invalid" id="validation-ex" placeholder="" value="" required/>
<div className="invalid-feedback"><i className="fa fa-exclamation-circle"/> Enter a last name</div>
</div>
</div>
<div className="form-group">
<label htmlFor="textArea1" className="col-sm-3 control-label">TextArea</label>
<div className="col-sm-12">
<textarea id="textArea1" className="form-control" rows="3" placeholder="Enter ..."/>
</div>
</div>
<div className="form-group">
<label htmlFor="select-1" className="col-sm-12 control-label">Select</label>
<div className="col-sm-offset-2 col-sm-12">
<select multiple="" id="select-1" className="form-control">
<option>option 1</option>
<option>option 2</option>
<option>option 3</option>
<option>option 4</option>
<option>option 5</option>
</select>
</div>
</div>
<div className="form-group">
<label htmlFor="check-1" className="col-sm-3 control-label">Checkbox</label>
<div className="col-sm-offset-2 col-sm-10">
<div className="form-check">
<input className="form-check-input" id="check-1" type="checkbox" value="option1"/>
<label className="form-check-label" htmlFor="check-1">Checkbox</label>
</div>
</div>
</div>
<div className="form-group">
<label htmlFor="radio-1" className="col-sm-3 control-label">Radio</label>
<div className="col-sm-offset-2 col-sm-10">
<div className="form-check">
<input className="form-check-input" id="radio-1" type="radio" value="option1"/>
<label className="form-check-label" htmlFor="radio-1">Radio</label>
</div>
</div>
</div>
<div className="form-group">
<label htmlFor="terms-1" className="col-sm-12 control-label">Terms and conditions</label>
<div className="col-sm-offset-2 col-sm-10">
<div className="form-check">
<input className="form-check-input is-invalid" type="checkbox" value="" id="invalidCheck3" required/>
<label className="form-check-label" htmlFor="invalidCheck3">
Agree to terms and conditions
</label>
<div className="invalid-feedback">
You must agree before submitting.
</div>
</div>
</div>
</div>
</div>
<div className="card-footer">
<Link href="/"><button type="submit" className="btn btn-info float-right">Save</button></Link>
</div>
</form>
</div>
</div>
</AdminLayoutHoc>
}
}
-EOF
Update styles.scss¶
cat <<'-EOF' > styles/styles.scss
/**
* App entry scss file
*
* @url https://getbootstrap.com/docs/4.0/getting-started/theming/
* @url https://getbootstrap.com/docs/4.0/getting-started/webpack/#importing-precompiled-sass
*/
//$blue: purple; // Override variables here to style accordingly
@import '~bootstrap/scss/bootstrap';
@import "~admin-lte/build/scss/AdminLTE-raw.scss";
$color: blue;
h1 {
color: $color;
}
.color-palette {
height: 35px;
line-height: 35px;
text-align: center;
}
-EOF